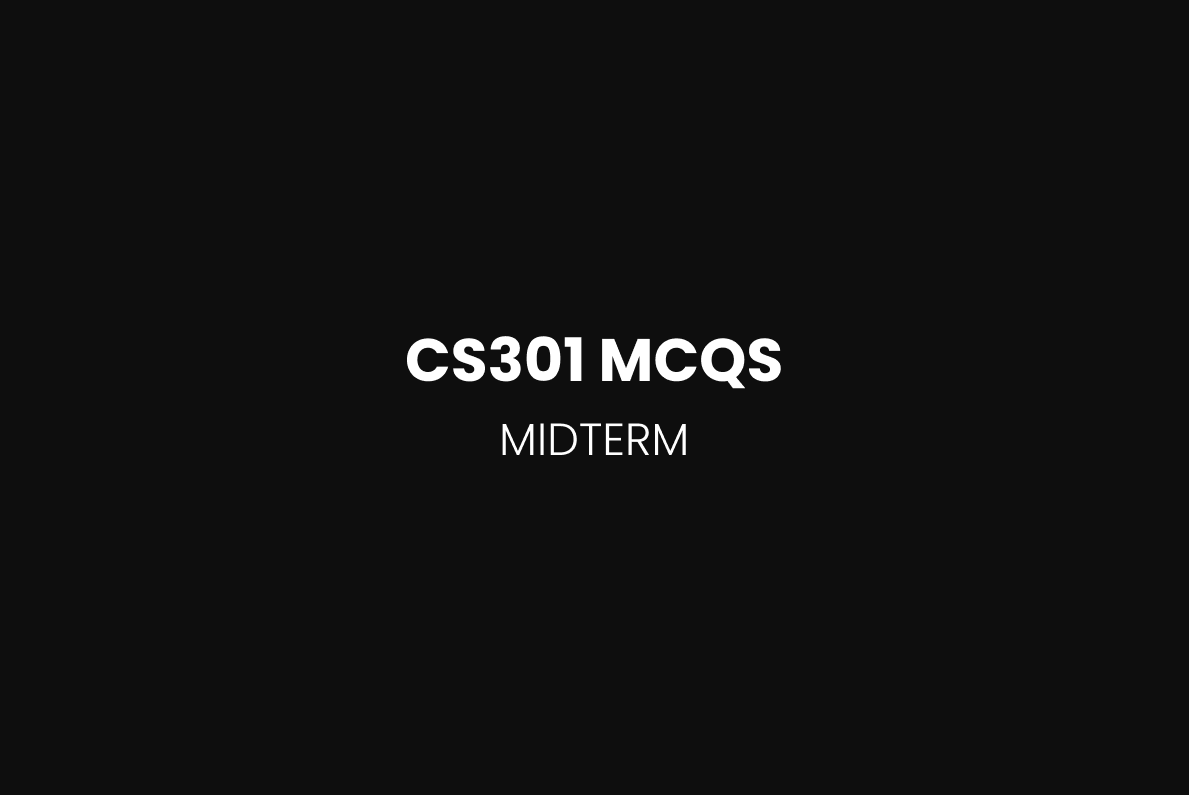
The provided document is a collection of MCQs for CS-301: Data Structures mid-term, designed to help students prepare effectively. Here’s a brief overview of the key topics and important concepts covered in the notes:
1. Basic Concepts of Data Structures:
- Array: The structure and behavior of arrays, such as accessing elements using index positions, and the characteristics of static and dynamic memory allocation.
- Stack: The document covers stack operations like push, pop, and top, emphasizing the Last In, First Out (LIFO) principle. Implementation of stacks using arrays or linked lists is also explored.
- Queue: Concepts of queue operations like enqueue and dequeue, which work on the First In, First Out (FIFO) principle. It also mentions circular queues and their operations.
- Linked Lists: Questions on singly linked lists, doubly linked lists, and circular linked lists, with explanations of node structure and pointer usage.
2. Tree Data Structures:
- Binary Search Tree (BST): Many questions focus on properties of binary trees, tree traversal methods (inorder, preorder, postorder), and node operations like insertion and deletion.
- AVL Tree: Concepts of AVL tree balancing with rotations (left, right, double rotations) are covered. The importance of maintaining height balance in AVL trees is emphasized.
- Tree Traversals: The document includes traversal methods like inorder, preorder, and postorder, asking students to identify or implement them in code.
3. Memory Management:
- Heap: The concept of the heap in memory, dynamic memory allocation, and deallocation using
new
anddelete
operators in C++ are discussed. - Stack Memory: Explanation of how function calls are managed using stack memory, including local variables, recursive calls, and the stack’s role in managing function execution.
4. Recursive Functions:
- Recursion: Many questions involve understanding recursive functions, their base conditions, and how recursion is implemented for tree traversals or factorial calculations.
5. Operations on Data Structures:
- Infix to Postfix Conversion: Conversion of expressions from infix to postfix using stacks is frequently asked. This includes precedence rules and operand handling.
- Postfix Evaluation: How to evaluate postfix expressions using stacks is also tested.
6. Pointers and Memory Addressing:
- Pointers: There are questions about pointer operations, pointer dereferencing, and their role in dynamic memory management, particularly in relation to linked lists and tree nodes.
7. Algorithms and Efficiency:
- Efficiency of Operations: Various MCQs focus on understanding the efficiency of operations in different data structures, such as searching and sorting in arrays, linked lists, and trees. There are questions related to time complexity and the most efficient data structures for specific tasks.
8. Special Data Structures:
- Circular Linked Lists: These are discussed for their efficiency in certain operations compared to singly or doubly linked lists.
- Simulation Models: The notes touch upon event-based and time-based simulation models in relation to data structures.
9. Expression Evaluation and Tree Properties:
- Expression Trees: There are problems involving the creation of trees from arithmetic expressions, including binary trees used to evaluate expressions.
- Binary Tree Properties: Questions about the properties of complete, full, and perfect binary trees, including their height, depth, and number of nodes.
10. Function Prototypes and Overloading:
- Function Overloading: The notes briefly discuss function overloading and signatures in C++, emphasizing the importance of understanding how different function types are declared and called.
These notes provide a comprehensive guide to fundamental concepts in data structures, especially for students preparing for mid-term exams. They emphasize theoretical understanding along with practical implementation through multiple-choice questions and problem-solving.
Leave a Reply