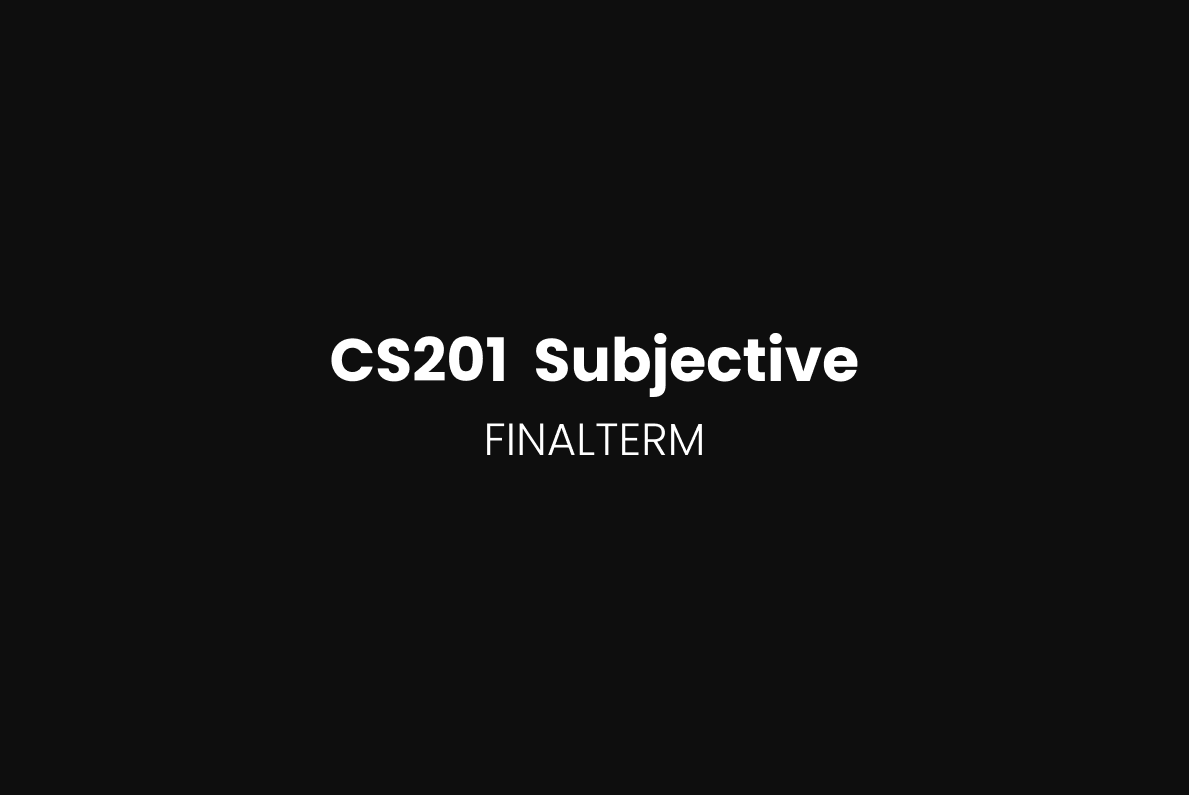
The document you’ve provided outlines important topics for the final exam of CS201, a course likely focused on Object-Oriented Programming (OOP) using C++. Below is a summary of key topics highlighted, which you can use for study notes:
1. Destructors and Constructors
- Destructor: Responsible for cleaning up memory when an object is destroyed.
- Constructor: Initializes objects. Types include default constructors, parameterized constructors, and copy constructors.
2. This Pointer
- A pointer in C++ that refers to the current object of a class, useful for distinguishing between object members and parameters.
3. Matrix Class & Operations
- Understanding of matrices, transpose, and operations such as matrix addition, multiplication, and overloading operators like
==
to compare matrices.
4. Memory Management
- Concepts of new and delete operators for dynamic memory allocation and deallocation.
- Static and dynamic memory allocation differences.
5. Templates
- Template Classes and Functions: Allow generic programming in C++.
- Advantages and disadvantages of templates and how to implement them for different data types.
6. Friend Classes and Functions
- Friend classes/functions: These can access the private and protected members of another class.
7. Operator Overloading
- Overloading different operators like
==
,+
,-
,++
,--
, and assignment=
operators. - Restrictions on which operators cannot be overloaded.
- Writing programs for function and operator overloading, such as pre-increment and post-increment.
- Insertion
<<
and extraction>>
operator overloading.
8. Function Overloading & Overriding
- Function Overloading: Same function name with different parameters.
- Function Overriding: Redefining base class methods in a derived class.
9. Memory Allocation
- How to allocate and deallocate memory arrays (2D arrays, pointers).
- What happens if memory is not properly deallocated.
10. Classes and Objects
- Class vs. Structure: Understanding the differences in terms of default access specifiers and memory allocation.
- The concept of objects as instances of classes.
11. Code Error Identification
- Identifying common programming errors, like syntax or logical issues in C++ programs.
12. Program Output Analysis
- Determining output for small code snippets, such as function calls, loops, and conditional statements.
- Examples like modifying variables through references and evaluating loops to predict the number of executions.
13. Precision and Formatting
- Using
setprecision()
andsetfill()
to control the output of floating-point numbers and other formatted outputs.
14. Pointer Manipulation
- Swapping values using pointers.
- Difference between pointer usage in structures and classes.
15. Date and Person Class
- A typical example of creating a Date class and integrating it into a Person class, with private data members and default constructors.
16. Macros
- Using macros like
SUM
to calculate values by passing variables, showcasing preprocessor directives.
17. Loop and Control Statements
- Analyzing how loops (for, while, etc.) work, along with conditions like
break
andcontinue
.
Conclusion
These topics encompass the key elements of C++ programming related to memory management, object-oriented concepts, operator overloading, and error handling. By mastering these areas, you can effectively answer subjective questions in the CS201 final exam. The document also includes example problems that require predicting outputs or identifying errors, providing practical scenarios to apply your knowledge.
Leave a Reply