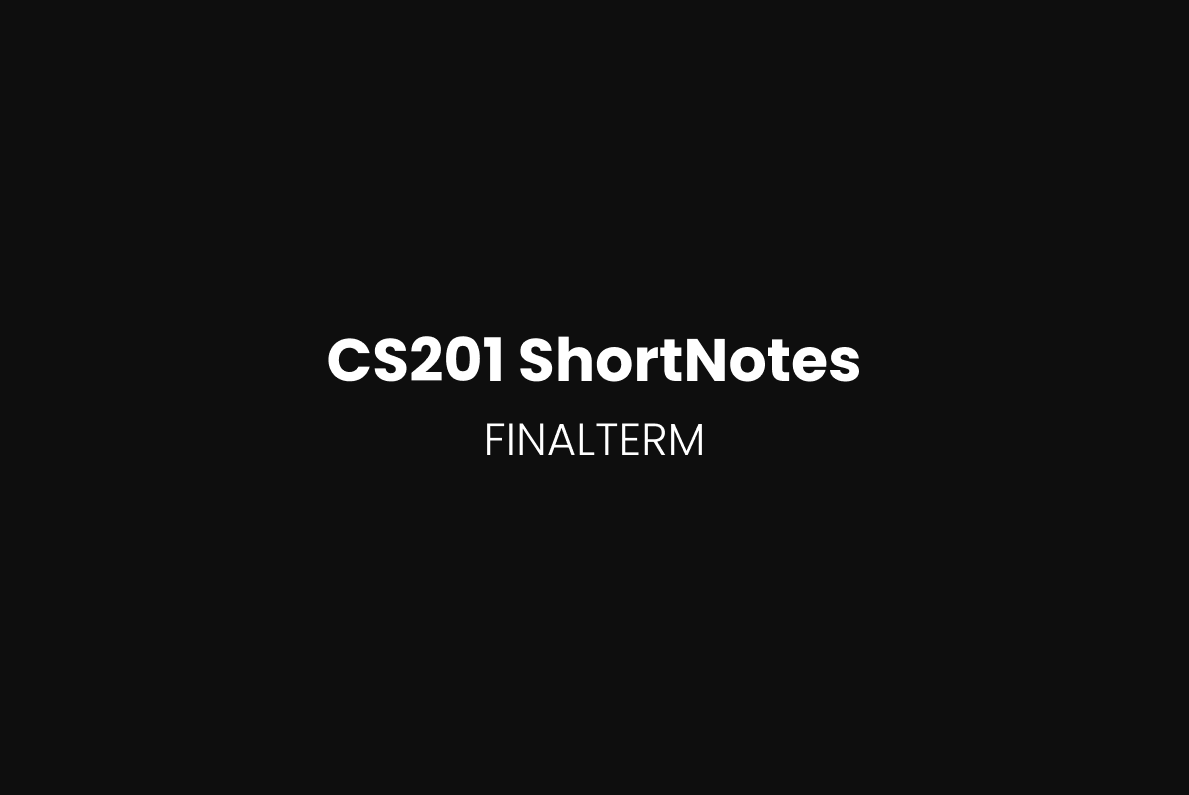
These notes serve as a comprehensive summary of key concepts in C++ programming, tailored for final term exam preparation. The document includes explanations of critical topics from basic programming constructs to advanced object-oriented programming (OOP) techniques. Here’s an overview of the important topics covered:
#include Directive:
The notes begin by explaining the#include
directive, which allows the inclusion of files into the source code. This is important for modular programming and code reuse. System-provided files are enclosed in angle brackets (< >
), while user-provided files are included using quotes.Abstract Classes and Inheritance:
Abstract classes, which serve as base classes for other classes, are defined. The importance of having at least one pure virtual function to make a class abstract is highlighted. Inheritance, a key OOP concept, is also covered, where derived classes inherit properties and behaviors from base classes.Memory Allocation:
Dynamic and static memory allocation are discussed. Concepts like thenew
anddelete
operators for dynamic memory management are explained, along with common issues like memory leaks. Additionally, the document touches on memory storage in C++ through terms like heap, stack, and pointers.Functions and Function Overloading:
The document explains how functions work in C++, including passing arguments by value and reference, function prototypes, and return types. The concept of function overloading, where multiple functions can share the same name but differ in parameter types, is also covered.Data Types and Keywords:
A detailed overview of C++ data types (likeint
,float
,char
, anddouble
) is provided. The importance of understanding the difference between signed and unsigned types is highlighted. Important keywords likebreak
,continue
,return
, and others are also explained.Object-Oriented Programming (OOP):
Several OOP principles are explained, such as encapsulation (using classes), inheritance (using base and derived classes), and polymorphism (using virtual functions). The role of constructors and destructors in object lifecycle management is also discussed.Data Structures:
Common data structures like arrays, linked lists, queues, and stacks are covered. The notes explain how data structures can help in organizing and managing data efficiently.Preprocessing and Macros:
The preprocessor’s role in handling macro expansion and code substitution is discussed. The document touches on how C++ provides features likeinline
functions that reduce the need for macros.Pointers and References:
There is a detailed explanation of how pointers work, including pointer arithmetic, pointers to functions, and memory management using pointers. The concept of references is also covered, explaining how they provide an alternative way to access objects without using pointers.Error Handling and Debugging:
Basic debugging concepts, like using debuggers to trace program execution, and common errors (e.g., memory leaks, segmentation faults) are discussed. Error handling techniques are briefly mentioned.Templates and Template Classes:
The document introduces the concept of templates, which allow writing generic code that works with any data type. This includes an explanation of template classes and functions.
In summary, these notes provide a strong foundation for understanding essential C++ programming concepts. They cover a wide range of topics, from basic syntax and data types to more advanced features like templates, memory management, and object-oriented design, making them ideal for a quick revision before exams.
Leave a Reply