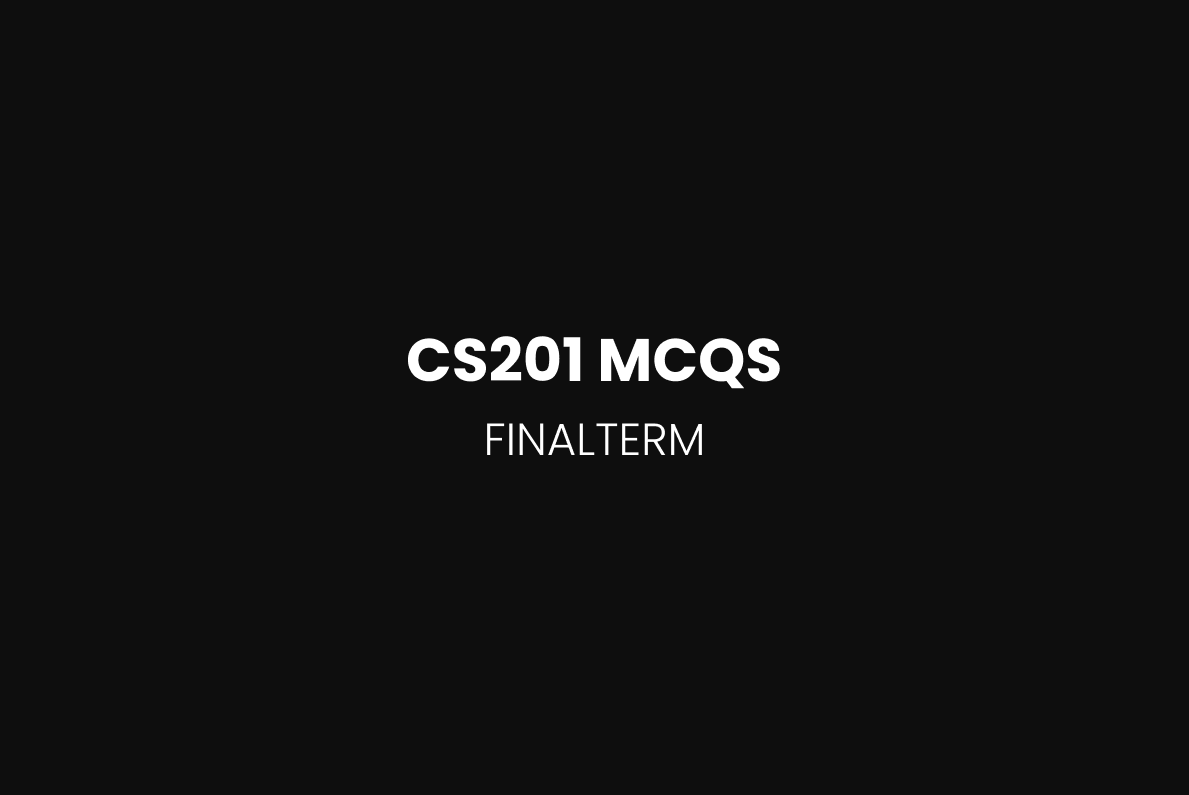
The notes from CS201 Final Term MCQs cover essential programming topics in C++, a powerful and widely used language in software development. Below is a description of the major areas and key topics addressed in the document:
1. Basic Programming Concepts:
- The document explores basic C++ programming concepts, including data types (e.g., integers, floats, pointers), memory allocation (using
malloc()
,realloc()
), and control structures. - Topics like function overloading, constructors, destructors, and static members are covered, providing a solid understanding of object-oriented programming principles.
2. Object-Oriented Programming (OOP):
- Core OOP concepts are thoroughly discussed, including classes, objects, inheritance, encapsulation, polymorphism, and operator overloading. For example, questions explain the role of constructors in initializing objects and destructors in memory management.
- Concepts like friend functions and their usage to bypass access restrictions are explored in depth, enhancing understanding of class relationships.
3. Memory Management:
- The document addresses memory allocation using the
new
anddelete
operators, as well as dynamic memory allocation through pointers and arrays. Topics like heap memory, stack memory, and memory leakage are also examined. - Key topics include the handling of memory using pointers and the implications of improper memory handling, such as dangling pointers and memory leaks.
4. Operator Overloading:
- There are several questions on operator overloading, explaining how operators like
+
,-
,++
, and=
can be customized for user-defined types. This is a critical concept in C++ that allows more flexible object manipulation.
5. File Handling:
- The notes touch on file handling in C++, covering input and output stream objects (such as
fstream
,ifstream
, andofstream
) and how to manage data from files. Functions likeseekg()
,tellg()
, andseekp()
are discussed, which allow for file navigation.
6. Pointers and References:
- Pointers and references are integral parts of C++, and the document covers their usage for dynamic memory allocation, function arguments, and object manipulation.
- Topics like null pointers, pointer arithmetic, and the difference between pointers and references are crucial for understanding memory management in C++.
7. Preprocessor Directives and Macros:
- Preprocessor directives like
#define
and#include
are covered, which allow for macro definitions and conditional compilation. This is important for writing efficient and portable C++ programs.
8. Error Handling and Debugging:
- Questions address error handling mechanisms in C++, such as catching and handling exceptions. Understanding the role of
try
,catch
, andthrow
is vital for writing robust programs.
9. Data Structures and Algorithms:
- The notes delve into basic data structures like arrays, structures, and unions. There’s also coverage of common algorithms, including sorting and searching, which are essential for problem-solving in programming.
10. Stream Manipulation and I/O:
- Stream manipulation using C++’s input/output facilities (
cin
,cout
) is covered, along with formatting options likesetprecision()
,setw()
, andendl
. These are necessary for handling user input and displaying output in a structured manner.
These topics form the backbone of programming in C++, equipping students with the skills necessary to develop efficient, scalable software systems. This guide is particularly useful for final-term exam preparation, offering a concise yet comprehensive review of the subject matter.
Leave a Reply